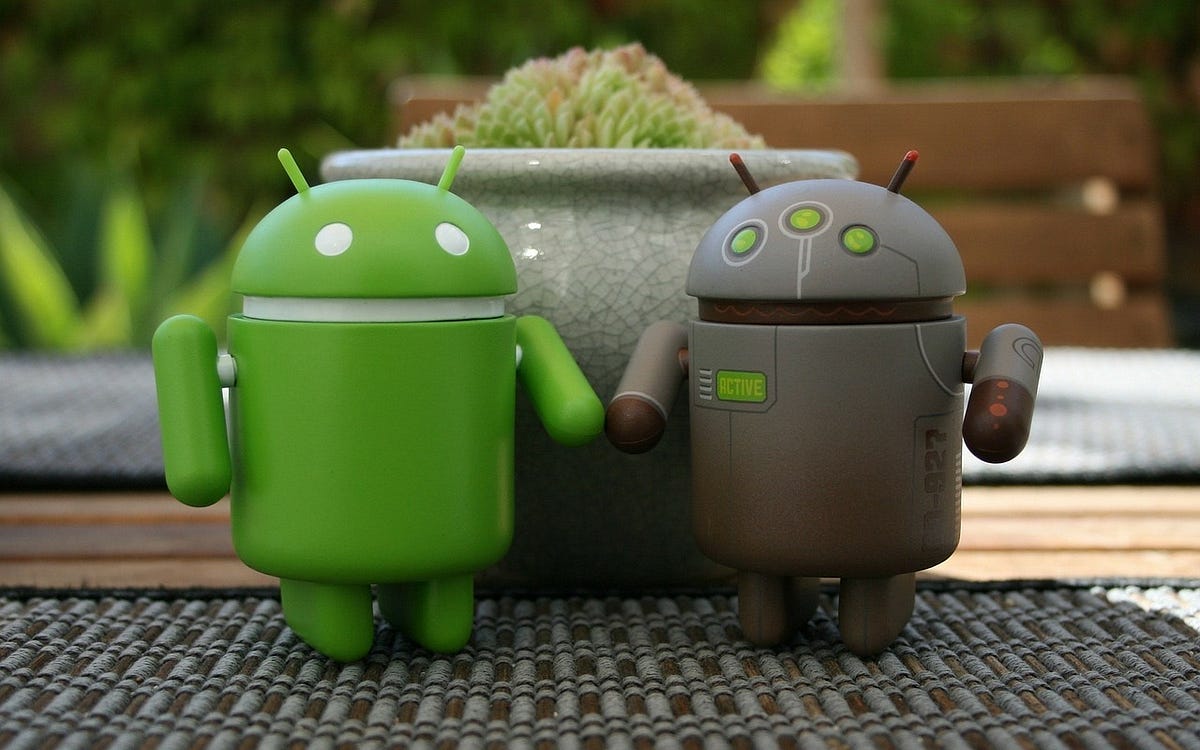
compile ‘android:best:1.1.1’
After reading lots of blogs, watching conferences/videos and from my own developing career I quickly came up with this list of libraries and resources that are probably used most often and approved by the community (also official ones from Google).
I am pretty sure there are hundreds of such lists compiled online (some of them at the end of this blog post). However, I would like to keep this relevant and up to date.
General goodness
More general stuff, that is not focused on one thing.
Android Developers Google+ Channel. Latest news from Android development world. Includes news from Android Developers Blog and Youtube channels.
Android Google+ Channel. Official news from Android for consumer world.
Android Weekly. Weekly newsletter with cutting edge information from Android Development. Must read. This website also features list of incoming conferences worldwide.
Android Backstage. Official podcast from Android developers, that’s has been on the air for a while. Interviews with people working on Android and Google platforms.
Fragmented. Another awesome podcast from Android Development world, interviews with developers, lots of resources and tips. Must listen.
Google I/O. Official annual conference from Google, which also covers all the latest news from Android world. If you can’t make it, you can watch talks on the official website or through official Google IO app.
AndroidDevSummit. Official annual (only one happened so far) conference from Google all about Android.
DroidCon. Annual Android development conference happening worldwide. Definitely, check it out. Again if you can’t make it, some of the talks are uploaded to youtube after (some of those that already happened in 2015): NYC, Berlin, Montreal, Spain, London.
Android Developers on Twitter. Official up to date tweets from Android devs.
Android Dialogs. Interviews with Android developers on their experience and good tips.
Londroid Meetup. Great talks happening in London all about Android. Talks from fellow developers and guests from Google. FREE.
Android Developers on Medium. A series of posts on Android Development from developers of Android includes good tips, practices, and guidelines on these topics:
- Introduction
- Mobile Context
- Memory
- Performance
- Networking
- Java and Data Structures
- Storage
- Framework and Patterns
- User Interface
- Tools
Android Studio. Official IDE for Android development, based on very popular IntelliJ platform. Official Eclipse IDE for Android development has been deprecated if you are still using it’s highly recommended to migrate to AS. Recently major version 2.0 was released (at the moment released in Canary channel, if you are not comfortable with it, you can install it side-by-side with the existing stable AS you have). One of the new features is new faster Android Emulator and a feature Instant Run, which will speed up APK deployment on device or emulator, it’s automatically turned on new projects, you can turn it on existing projects:
Settings/Preferences -> Build, Execution, Deployment -> Instant Run -> Enable Instant Run…
Also some good Android Studio configuration tips.
Also, must watch talk on Android Studio For Experts from Android Dev Summit 2015, very dense in information and tips.
Genymotion Emulator. 3rd party emulator, which is known for great performance and abilities to emulate various hardware features. Also has Gradle plugin, that allows controlling devices from gradle script. Free only for non-commercial use.
Support Library from Google delivers new APIs and stability fixes to older versions of Android. There are several versions that have particular minimum SDK version, some of them:
compile ‘com.android.support:support-v4:x.y.z’
compile ‘com.android.support:appcompat-v7:x.y.z’
compile ‘com.android.support:support-v13:x.y.z’
...
Make sure you update Android Support Library with SDK Manager. As those by default are pulled to project from local files rather than cloud repository.
Even if you target high API level devices it is still recommended to use a support library as they are updated and fixed constantly rather than being bound to device specific version (recommended usage is v4+v7-appcompat). Also, it’s not recommended to mix support and non-support APIs, even if they would compile, they can bring instabilities and even crashes.
Google Play Services provides various services and APIs from Google from Google Maps to Android Pay, from Wearables to Ads. It is updated from Google Play Store so it’s Android OS version independent. To include in your project:
compile ‘com.google.android.gms:play-services:x.y.z’
If you don’t want to include the whole package (as it is very big and you might run into over 65k method error), you can selectively include modules you want to use like:
compile ‘com.google.android.gms:play-services-wearable:x.y.z’
compile ‘com.google.android.gms:play-services-maps:x.y.z’
...
RxJava is a Java VM implementation of Reactive Extensions, helps you to compose asynchronous, event-based, stream like code and adds functional programming paradigm. Say good-bye to AsyncTasks.
compile ‘io.reactivex:rxjava:x.y.z’
RxAndroid wraps RxJava library. It adds functionality around Android specific threading. Note RxAndroid automatically includes a version of RxJava. However, because RxAndroid releases are behind it and if you want to use latest RxJava, then include both like:
compile ‘io.reactivex:rxandroid:x.y.z’
compile ‘io.reactivex:rxjava:a.b.c’
RxLifecycle adds automatic unsubscription from sequences based on Activity/Fragment. Useful as incomplete subscriptions can cause memory leaks same as:
compile ‘com.trello:rxlifecycle:x.y.z’
compile ‘com.trello:rxlifecycle-components:x.y.z’
RxBinding provides binding APIs for Android UI widgets from the platform and support libraries.
compile ‘com.jakewharton.rxbinding:rxbinding:x.y.z’
compile 'com.jakewharton.rxbinding:rxbinding-support-v4:x.y.z'
...
Learning Reactive Extensions is a ‘bit’ of a learning curve, but once you get it, you won’t come back. Learn more about it here:
- Official site
- Interactive diagrams of Rx Observables
- Replace AsyncTask with RxJava (blog post)
- Grokking RxJava (blog post)
- Fragmented episodes 3 and 4 (podcast)
- Advanced RxJava (blog post)
- RxJava for Android App Development (free ebook)
Frodo. Plugin, which helps you to log RxJava subscribers and observables inputs and outputs.
classpath 'com.fernandocejas.frodo:frodo-plugin:x.y.z'
apply plugin: 'com.fernandocejas.frodo'
IOSched application. The source code of Google I/O Android App. Showcases latest development features and trends from Android developers.
u2020 Application. A good example of Android application, “which showcases advanced usage of Dagger among other open source libraries” (mostly from Square).
Commonsware Book. Online book about Android development, which contains large amount of topics. Book itself is constantly updated. Price: 45$ per year.
Code OCD
Libraries that helps to keep your code cleaner.
Support Annotations. Library from Google that includes a number of useful annotations like @Nullable, @StringRes etc to help catch bugs and make your code more clear to yourself and your team. Blog post on tips how to use these annotations.
compile ‘com.android.support:support-annotations:x.y.z’
Retrolambda Gradle Plugin. Introduces Java 8 Lambdas to Java 6/7 (as well as Android). Makes your code much cleaner, combine it with RxJava and you are solid. Say goodbye to callback hells.
classpath 'me.tatarka:gradle-retrolambda:x.y.z'
apply plugin: 'me.tatarka.retrolambda'
Butterknife. Provides annotations for view finding, view holders (for recycle view/list view) and view events (OnClick, etc). Forget countless lines and casts like (TextView) context.findViewById(R.id.blah)…
compile ‘com.jakewharton:butterknife:x.y.z’
Also, there is a great Android Studio plugin that helps you automatically generate bindings.
Data Binding Library. Library (more like a plugin) from Google that is still in beta. Allows automatic data binding in layout XML files rather than in java reducing boilerplate code. Requires Android Plugin for Gradle 1.30-beta4 or higher. To learn more read on these blog post series. Also, check this tip how to apply custom font in XML using this library! To enable this plugin:
android {
....
dataBinding {
enabled = true
}
}
Dagger 2. Library started by Square, now developed by Google too. Android platform suffers from tight coupling with system components and it’s hard to inject dependencies (think of Activities, Fragments, Services, Loaders, etc). This library adds ‘dependency injection design pattern without the burden of writing boilerplate’. Allows to separate your code into more meaningful modules and manage dependencies using dependency graph. Makes your code more reusable, testable and (arguably) readable.
compile ‘com.google.dagger:dagger:x.y.z’
Parcelable Generator. This plugin generates that Parcelable boilerplate for you.
Building…
Building and compiling related stuff.
Multidex Support Library. If you ever run into ‘Over 65k Methods’ Error while you build your app, this might be your cure (however this should be a last resort, you should try to strip it down your app first):
compile ‘com.android.support:multidex:x.y.z’
Don’t forget to set multiDexEnabled true in your defaultConfig gradle configuration and extend your Application class to MultiDexApplication.
ReLinker. Native library loader for Android, which replaces standard System.loadLibrary to fix infamous UnsatisfiedLinkError.
compile 'com.github.KeepSafe:ReLinker:x.y'
Library Publishing. A step by step tutorial on publishing your library to Maven repo (also check out blog posts at the end).
TDD
Or just everything that helps you test your code.
Testing Support Library. Official test framework from Google for Android. Setup examples can be found here. You can have 2 kinds of tests:
- Pure JUnit tests
Runs on JVM. Does not require device or emulator. See ‘Plain Java module’ example. Usually fast (most suitable for TDD). This is where your domain/business logic should be. You only need to include JUnit test library:
testCompile ‘junit:junit:4.x’
Also, note before to be able to run those tests, your code couldn’t have any dependencies on Android classes. Since gradle plugin version 1.1 mocked Android.jar (no actual code) is provided, so you are able to have Android classes as long as you don’t execute methods of those classes. If you are still using them, then you must use a mocking library like Mockito. To run JVM tests with Android APIs you can use Robolectric library (described below).
- Instrumentation tests
Runs on device/emulator. Can be very slow (not suitable for TDD). Can be used for functional/UI tests. You can interact with Android framework: activities, views, context, etc… To run those tests you would need to include Test Runner library:
androidTestCompile ‘com.android.support.test:runner:x.y’
Also, specify runner in defaultConfig gradle configuration:
testInstrumentationRunner “android.support.test.runner.AndroidJUnitRunner”
Most of the cases in your functional test you would need to extend ActivityInstrumentationTestCase2, which will provide functional testing of a single activity.
For testing views, Test Support Library also includes Espresso library (it’s being moved out from android-test-kit), which has a very subtle API (Hamcrest matcher based, which is also included). It also can wait for asynchronous operations. To include:
androidTestCompile ‘com.android.support.test.espresso:espresso-core:x.y.z’
If you need to mock callback from Intent, then you can use Espresso Intents library:
androidTestCompile ‘com.android.support.test.espresso:espresso-intents:x.y’
If you need to run instrumentation tests across different activities (even from system or external apps), you can use UiAutomator:
androidTestCompile ‘com.android.support.test.uiautomator:uiautomator-v18:x.y.z’
Also, if you want to use JUnit4 rules on your instrumentation tests like @Test, @Before, etc… you would need to include Test Rules library:
androidTestCompile ‘com.android.support.test:rules:x.y’
See examples page how to run (JVM or Instrumentation) both from a command line and Android Studio GUI. Also, take a look at practices for testing.
Robolectric allows running Android tests on JVM fast (without device/emulator). Robolectric rewrites Android SDK jar classes by ‘shadowing’ them (which kinda makes testing Robolectric rather than Android), it has a bit of a learning curve and sometimes it can be tricky setting it up. But once you get it, you don’t need to rely on instrumentation tests and not need to worry too much about separating classes to pure and non-pure java for TDD, etc. If it’s a good thing or not, that’s for you to decide:
testCompile ‘org.robolectric:android-all:x.y.z’
Hamcrest provides matchers for your tests. You can combine, filter test results more efficiently and it’s more readable:
testCompile ‘org.hamcrest:hamcrest-junit:x.y.z’
Mockito. Mocking framework for unit tests in Java. Provides clean and simple API:
testCompile ‘org.mockito:mockito-all:x.y.z’
Everything that’s pretty
Views, UI, Design, etc…
Design Support Library brings back Lollipop UI goodness to older versions of Android like NavigationBar, Tabs, SnackBar, FAB, CoordinatorLayout (also this blog post), etc.
compile ‘com.android.support:design:x.y.z’
Read more about it on a blog post. Samples from Google.
RecyclerView is de facto new ListView. RecyclerView provides better API for customizing your views that need to be recycled (like lists while scrolling…). Also automatically uses ViewHolder pattern (which needs to be manually implemented on ListView). Also has built in functionality with CoordinatorLayout. More about it here.
compile ‘com.android.support:recyclerview-v7:x.y.z’
That doesn’t mean you have to replace all your ListViews in code (it’s not deprecated), but for the new stuff you should consider using it as it is most likely to be updated by Android team.
Material Design Guidelines from Google very useful both for developers and designers. This spec is being constantly updated.
Material Design for Android Developers Course. Free course by Android Developers for Android Developers. All you need to know about Material Design and animations and how to implement it. 4 weeks course. Highly recommended.
Material Design Blog Posts. A collection of great articles about Material Design implementations.
- Material Design Checklist (from official blog)
- Implementing Material Design (from official blog)
- Activity And Fragment Transitions series
- Exploring Meaningful Motion
Material Icons. Official icons to use for your Material Design app. Both in vector and png formats.
Topeka application. Application, that showcases material design on Android (open source).
Plaid application. More recent application to showcase material design (open source).
Android Experiments. Web site, that is designed to showcase various Android experiments from both creativity and code perspectives. Get inspired and submit yours!
Data 101
Libraries that handles your data.
Picasso/Glide/Fresco. Excellent and efficient image loading libraries from network and more.
Picasso from Square. Very simple API. Works with OkHttp.
compile ‘com.squareup.picasso:picasso:x.y.z’
Glide from BumpTech. Similar API to Picasso, but more expandable, also claims to be faster. Can work with OkHttp or Volley.
compile ‘com.github.bumptech.glide:glide:x.y.z’
Fresco from Facebook. Even more expandable. Claims to be fastest (using native c layer). Big in size (consider using it if your app is very media focused).
compile ‘com.facebook.fresco:fresco:x.y.z’
Read through what features those libraries provide and decide which one to use for your app.
Retrofit. If you need to communicate to Rest API from your app (probably 90% of apps), then this library from Square is for you. Very simple and clean annotation based API. Can automatically convert JSON to POJOs and vice versa (compatible with Gson, Jackson, Protocol Buffers, etc…). Compatible with RxJava. Compatible with OkHttp.
compile ‘com.squareup.retrofit:retrofit:x.y.z’
It was recently updated to version 2, check this presentation (and video link in description) to see how to use it.
OkHttp. Http client by Square. Solves various network connection issues. Also different versions of Android may use different versions of http and can have different implementations, by using this you can be ensured your connections on different version will work the same.
compile ‘com.squareup.okhttp:okhttp:x.y.z’
Gson. Very popular JSON data parsing library by Google. Can be used to convert JSON to Java objects and vice versa.
compile ‘com.google.code.gson:gson:x.y.z’
Protocol Buffers. A language-neutral, platform-neutral extensible mechanism for serializing structured data by Google. Alternative to JSON.
DiskLruCache. If you ever need to use disk caching besides existing memory cache API, you can use this. It’s being used in Android platform itself.
compile ‘com.jakewharton:disklrucache:x.y.z’
Note image loading libraries mentioned above already has caching implemented. More about caching on Android.
Android Joda Time. Joda Time solves lots of issues currently with Java’s Date and Calendar classes. So if you need a better date/time data management, this library is for you. This is Joda Time port optimized for Android platform.
compile ‘net.danlew:android.joda:x.y.z’
Assistance
Helps to debug your application.
Stetho. Powerful debug tool from Facebook for your Android app. Allows to inspect network requests/responses, databases (SQLite + preferences), view hierarchy and more by using Chrome DevTools.
compile ‘com.facebook.stetho:stetho:x.y.z’
To use tool with okhttp or urlconnection respectively:
compile ‘com.facebook.stetho:stetho-okhttp:x.y.z’
compile ‘com.facebook.stetho:stetho-urlconnection:x.y.z’
LeakCanary. If you ever run into dreadful OOM (out of memory error), then this library from Square is for you. After setting up LeakCanary, when a leak happens, it will show a notification, which gives insightful information about it.
debugCompile ‘com.squareup.leakcanary:leakcanary-android:x.y.z’
To learn more about it on this blog post.
Go Live!
Everything related to publishing your app and Google Play Store
Tips for Success on Google Play. Series of videos on tips and ways to achieve your app goals on Play Store. Also recently book featuring Google Play possibilities and similar tips was released (free).