初始化项目
- 创建文件:mkdir logmei-js-demo
- 初始化package.json:npm init
- 安装插件:
npm install --save-dev typescript ts-loader webpack
- 配置tsconfig.json
//tsconfig.json
{
"compilerOptions": {
"outDir": "./dist/",
"noImplicitAny": true,
"allowJs": true,
"module": "es6",
"target": "es5"
},
"exclude": ["node_modules"],
"include": ["src/*"]
}
- 配置webpack.config.js
const path =require('path');
module.exports = {
mode:'development',
entry:'./src/index.ts',
devtool:'cheap-source-map',
output:{
filename:'bundle.js',
path:path.resolve(__dirname,'dist')
},
module:{
rules:[
{
test:/\.tsx?$/,
use:'ts-loader',
exclude:/node_modules/
}
]
},
resolve: {
extensions:['.tsx','.ts','.js']//
}
}
- 配置package.json
//package.json
{
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"build": "webpack --config webpack.development.conf.js"
}
}
- 编写ts文件,并测试
//test.ts
let test:string = 'logmei'
console.log(test)
运行tsc命令,成功生成js文件
tsc src/test.ts
- 运行命令:npm run build;编译完成
本地运行服务
- 添加index.html模板
- 安装插件html-webpack-plugin,动态生成html,添加配置
安装插件
npm i html-webpack-plugin -D
//webpack.development.conf.js
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
plugins:[
new HtmlWebpackPlugin({
template:'./public/index.html'
})
]
}
- 添加本地运行环境和热启动
安装插件
npm i webpack-dev-server -D
//package.json
{
"scripts": {
"dev": "webpack-dev-server --open --config webpack.development.conf.js"
}
}
//webpack.development.conf.js
const webpack = require('webpack');
module.exports = {
plugins:[
new webpack.HotModuleReplacementPlugin()
],
devServer:{
port:8008,
open:true,
hot:true,
contentBase:'./dist'
}
}
运行命令:npm run dev启动服务
若报错如:
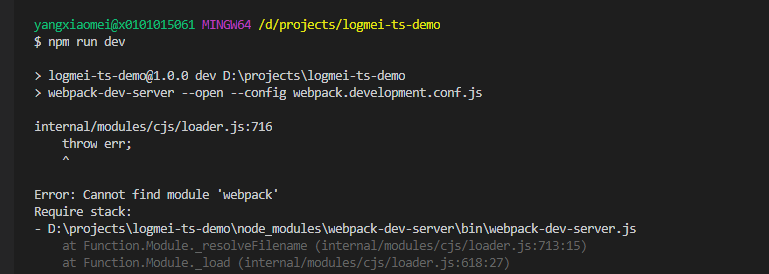
tsconfig的配置说明
tsconfig
属性值 | 描述 | 值类型 | 默认值 |
---|---|---|---|
compilerOptions | 编译配置 | Array | 官方默认配置 |
files | 由ts管理的具体路径 | Array | |
exclude | 排除的文件 | Array | |
include | 需要管理的文件 | Array | |
compileOnSave | 用于IDE保存时,是否生成编译后的文件 | Boolean | |
typeAcquisition | 设置自动引入库类型定义文件(.d.ts) | Object |
typeAcquisition
属性值 | 描述 | 值类型 | 默认值 |
---|---|---|---|
enable | 是否开启自动引入 | Boolean | false |
include | 自动引入库名 | Array | |
exclude | 排除库名 | Array |
compilerOptions
属性值 | 描述 | 值类型 | 默认值 |
---|---|---|---|
noImplicitAny | 不允许隐式 any,如果true,函数的形参必须带类型,如果叫不出class名的js对象,那就得any,比如(d:any)=>{},如果false,函数的样子更像js (d)=>{} | Boolean | false |
module | 模块生成方式 | String | target === "ES3" or "ES5" ? "CommonJS" : "ES6" |
target | 输出代码es版本["ES3","ES5","ES6"/"ES2015","ES2017","ES2018","ES2019" ,"ES2020","ESNext"] | String | ES3 |
allowJs | 编译时允许有js文件 | Boolean | false |
webpack配置
resolve
- alias:通过别名来把原来导入路径映射成一个新的导入路径
resolve:{
alias:{
@:'./src/'
}
}
- extensions:在导入语句没带文件后缀时,Webpack会自动带上后缀去尝试访问文件是否存在,resolve.extensions用于配置后缀列表
resolve:{
extensions:['.ts','.js']
}