Apple在iOS 6中添加了UIRefreshControl
,但只能在UITableViewController
中使用,不能在UIScrollView
和UICollectionView
中使用。
iOS 10 新特性
从iOS 10开始,UIScrollView
增加了一个refreshControl
属性,用于把配置好的UIRefreshControl
赋值给该属性,这样UIScrollView
就有了下拉刷新功能。和之前在UITableViewController
中使用一样,不需要设置UIRefreshControl
的frame
,只需要配置UIRefreshControl
。
因为UITableView
和UICollectionView
继承自UIScrollView
,所以UITableView
和UICollectionView
也继承了refreshControl
属性,也就是可以很方便的把刷新控件添加到滚动视图、集合视图和表视图(不再需要表视图控制器)。
截止目前,Xcode 8.2.1的Interface Builder还没有支持
refreshControl
属性,如果你需要在UIScrollView
、UITableView
和UICollectionView
中使用UIRefreshControl
只能通过代码添加。通过Interface Builder可以为UITableViewController
添加刷新控件。
滚动视图示例
这个demo使用Single View Application模板,打开storyboard,在系统创建的ViewController
上添加一个UIScrollView
,在UIScrollView
上添加两个UILabel
,并在UILabel
上添加内容。想要实现的功能是,下拉刷新页面时隐藏第二个UILabel
,再次刷新时显示该UILabel
。
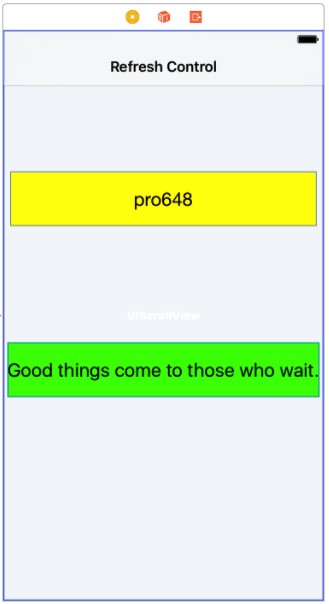
这里只对demo简单描述,如果需要查看详细代码,可以在我的GitHub中查看。另外,文章底部也会提供源码地址。
创建刷新控件
在UIScrollView
、UITableView
和UICollectionView
中创建刷新控件步骤是一样的。在这个示例中,在ViewController
的viewDidLoad
方法中创建并配置UIRefreshControl
。scrollView
是连接到Interface Builder中的UIScrollView
的IBOutlet属性。
- (void)viewDidLoad
{
[super viewDidLoad];
// 1 先判断系统版本
if ([NSProcessInfo.processInfo isOperatingSystemAtLeastVersion:(NSOperatingSystemVersion){10,0,0}])
{
// 2 初始化
UIRefreshControl *refreshControl = [[UIRefreshControl alloc] init];
// 3.1 配置刷新控件
refreshControl.tintColor = [UIColor brownColor];
NSDictionary *attributes = @{NSForegroundColorAttributeName : [UIColor redColor]};
refreshControl.attributedTitle = [[NSAttributedString alloc] initWithString:@"Pull To Refresh" attributes:attributes];
// 3.2 添加响应事件
[refreshControl addTarget:self action:@selector(refresh:) forControlEvents:UIControlEventValueChanged];
// 4 把创建的refreshControl赋值给scrollView的refreshControl属性
self.scrollView.refreshControl = refreshControl;
}
}
注意以下几点:
UIScrollView
从iOS 10开始才有refreshControl
属性,所以第一步判断当前系统版本。- 初始化刷新控件。
UIKit
会自动设置frame
,不需要手动设定。 - 3.1 配置刷新控件,可以通过
tintColor
设置进度滚轮指示器颜色,通过attributedTitle
添加刷新时显示的提示文字。3.2 添加响应事件,当UIControlEventValueChanged
事件发生时指定响应的动作。 - 把上面创建、配置的
refreshControl
赋值给scrollView
的refreshControl
属性
现在实现动作方法。available
是在interface部分声明的BOOL
类型的对象。
- (void)refresh:(UIRefreshControl *)sender
{
self.available = ! self.available;
self.secondLabel.hidden = self.available;
// 停止刷新
[sender endRefreshing];
}
如果secondLabel
目前显示,下拉后隐藏,如果目前隐藏,下拉后显示。最后使用endRefreshing
停止刷新。
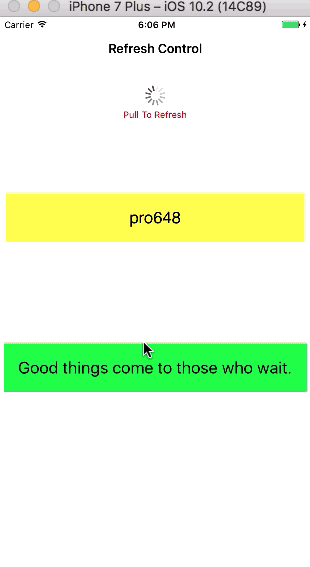
Demo名称:RefreshControl
源码地址:github.com/pro648/Basi…
参考资料: