基于el-slider自定义range组件封装实践
前言
日常工作中经常使用范围选择组件,例如进度条、日期范围选择等组件,常见组件库中经常使用的都是使用的圆形滑块形状,项目中有需要根据自定义去实现一个range组件,以下是基于element的el-slider组件进行改良封装的一个range组件。
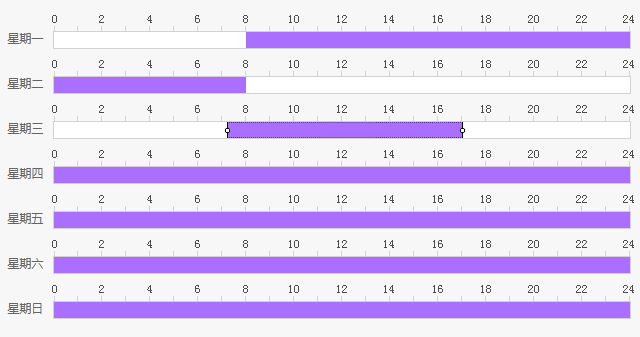
问题目录
- el-slider源码解读
- 自定义封装
- 封装range组件常见方法
探索案例
el-slider源码解读

[组件目录]
- src
- button.vue
- main.vue
- marker.js
- index.js
[目录描述] button主要是滑块的大小、样式,拖拽行为等方法的主体;main主要是进度条的显示
[源码分析] el-slider的主体是button和bar,在button上主要需要考虑的是事件相关的处理,其中包括mouseenter、mouseleave、mousemove、mouseup、mousedown、touchstart、touchuend、touchmove、keydown.left/right/down/up;bar上主要考虑的就是值的获取与位置的显示
button源码:
<template>
<div
class="el-slider__button-wrapper"
@mouseenter="handleMouseEnter"
@mouseleave="handleMouseLeave"
@mousedown="onButtonDown"
@touchstart="onButtonDown"
:class="{ 'hover': hovering, 'dragging': dragging }"
:style="wrapperStyle"
ref="button"
tabindex="0"
@focus="handleMouseEnter"
@blur="handleMouseLeave"
@keydown.left="onLeftKeyDown"
@keydown.right="onRightKeyDown"
@keydown.down.prevent="onLeftKeyDown"
@keydown.up.prevent="onRightKeyDown"
>
<el-tooltip
placement="top"
ref="tooltip"
:popper-class="tooltipClass"
:disabled="!showTooltip">
<span slot="content">{{ formatValue }}</span>
<div class="el-slider__button" :class="{ 'hover': hovering, 'dragging': dragging }"></div>
</el-tooltip>
</div>
</template>
<script>
import ElTooltip from 'element-ui/packages/tooltip';
export default {
name: 'ElSliderButton',
components: {
ElTooltip
},
props: {
value: {
type: Number,
default: 0
},
vertical: {
type: Boolean,
default: false
},
tooltipClass: String
},
data() {
return {
hovering: false,
dragging: false,
isClick: false,
startX: 0,
currentX: 0,
startY: 0,
currentY: 0,
startPosition: 0,
newPosition: null,
oldValue: this.value
};
},
computed: {
disabled() {
return this.$parent.sliderDisabled;
},
max() {
return this.$parent.max;
},
min() {
return this.$parent.min;
},
step() {
return this.$parent.step;
},
showTooltip() {
return this.$parent.showTooltip;
},
precision() {
return this.$parent.precision;
},
currentPosition() {
return `${ (this.value - this.min) / (this.max - this.min) * 100 }%`;
},
enableFormat() {
return this.$parent.formatTooltip instanceof Function;
},
formatValue() {
return this.enableFormat && this.$parent.formatTooltip(this.value) || this.value;
},
wrapperStyle() {
return this.vertical ? { bottom: this.currentPosition } : { left: this.currentPosition };
}
},
watch: {
dragging(val) {
this.$parent.dragging = val;
}
},
methods: {
displayTooltip() {
this.$refs.tooltip && (this.$refs.tooltip.showPopper = true);
},
hideTooltip() {
this.$refs.tooltip && (this.$refs.tooltip.showPopper = false);
},
handleMouseEnter() {
this.hovering = true;
this.displayTooltip();
},
handleMouseLeave() {
this.hovering = false;
this.hideTooltip();
},
onButtonDown(event) {
if (this.disabled) return;
event.preventDefault();
this.onDragStart(event);
window.addEventListener('mousemove', this.onDragging);
window.addEventListener('touchmove', this.onDragging);
window.addEventListener('mouseup', this.onDragEnd);
window.addEventListener('touchend', this.onDragEnd);
window.addEventListener('contextmenu', this.onDragEnd);
},
onLeftKeyDown() {
if (this.disabled) return;
this.newPosition = parseFloat(this.currentPosition) - this.step / (this.max - this.min) * 100;
this.setPosition(this.newPosition);
this.$parent.emitChange();
},
onRightKeyDown() {
if (this.disabled) return;
this.newPosition = parseFloat(this.currentPosition) + this.step / (this.max - this.min) * 100;
this.setPosition(this.newPosition);
this.$parent.emitChange();
},
onDragStart(event) {
this.dragging = true;
this.isClick = true;
if (event.type === 'touchstart') {
event.clientY = event.touches[0].clientY;
event.clientX = event.touches[0].clientX;
}
if (this.vertical) {
this.startY = event.clientY;
} else {
this.startX = event.clientX;
}
this.startPosition = parseFloat(this.currentPosition);
this.newPosition = this.startPosition;
},
onDragging(event) {
if (this.dragging) {
this.isClick = false;
this.displayTooltip();
this.$parent.resetSize();
let diff = 0;
if (event.type === 'touchmove') {
event.clientY = event.touches[0].clientY;
event.clientX = event.touches[0].clientX;
}
if (this.vertical) {
this.currentY = event.clientY;
diff = (this.startY - this.currentY) / this.$parent.sliderSize * 100;
} else {
this.currentX = event.clientX;
diff = (this.currentX - this.startX) / this.$parent.sliderSize * 100;
}
this.newPosition = this.startPosition + diff;
this.setPosition(this.newPosition);
}
},
onDragEnd() {
if (this.dragging) {
/*
* 防止在 mouseup 后立即触发 click,导致滑块有几率产生一小段位移
* 不使用 preventDefault 是因为 mouseup 和 click 没有注册在同一个 DOM 上
*/
setTimeout(() => {
this.dragging = false;
this.hideTooltip();
if (!this.isClick) {
this.setPosition(this.newPosition);
this.$parent.emitChange();
}
}, 0);
window.removeEventListener('mousemove', this.onDragging);
window.removeEventListener('touchmove', this.onDragging);
window.removeEventListener('mouseup', this.onDragEnd);
window.removeEventListener('touchend', this.onDragEnd);
window.removeEventListener('contextmenu', this.onDragEnd);
}
},
setPosition(newPosition) {
if (newPosition === null || isNaN(newPosition)) return;
if (newPosition < 0) {
newPosition = 0;
} else if (newPosition > 100) {
newPosition = 100;
}
const lengthPerStep = 100 / ((this.max - this.min) / this.step);
const steps = Math.round(newPosition / lengthPerStep);
let value = steps * lengthPerStep * (this.max - this.min) * 0.01 + this.min;
value = parseFloat(value.toFixed(this.precision));
this.$emit('input', value);
this.$nextTick(() => {
this.displayTooltip();
this.$refs.tooltip && this.$refs.tooltip.updatePopper();
});
if (!this.dragging && this.value !== this.oldValue) {
this.oldValue = this.value;
}
}
}
};
</script>
main源码:
<template>
<div
class="el-slider"
:class="{ 'is-vertical': vertical, 'el-slider--with-input': showInput }"
role="slider"
:aria-valuemin="min"
:aria-valuemax="max"
:aria-orientation="vertical ? 'vertical': 'horizontal'"
:aria-disabled="sliderDisabled"
>
<el-input-number
v-model="firstValue"
v-if="showInput && !range"
class="el-slider__input"
ref="input"
@change="emitChange"
:step="step"
:disabled="sliderDisabled"
:controls="showInputControls"
:min="min"
:max="max"
:debounce="debounce"
:size="inputSize">
</el-input-number>
<div
class="el-slider__runway"
:class="{ 'show-input': showInput, 'disabled': sliderDisabled }"
:style="runwayStyle"
@click="onSliderClick"
ref="slider">
<div
class="el-slider__bar"
:style="barStyle">
</div>
<slider-button
:vertical="vertical"
v-model="firstValue"
:tooltip-class="tooltipClass"
ref="button1">
</slider-button>
<slider-button
:vertical="vertical"
v-model="secondValue"
:tooltip-class="tooltipClass"
ref="button2"
v-if="range">
</slider-button>
<div
class="el-slider__stop"
v-for="(item, key) in stops"
:key="key"
:style="getStopStyle(item)"
v-if="showStops">
</div>
<template v-if="markList.length > 0">
<div>
<div
v-for="(item, key) in markList"
:style="getStopStyle(item.position)"
class="el-slider__stop el-slider__marks-stop"
:key="key">
</div>
</div>
<div class="el-slider__marks">
<slider-marker
:mark="item.mark" v-for="(item, key) in markList"
:key="key"
:style="getStopStyle(item.position)">
</slider-marker>
</div>
</template>
</div>
</div>
</template>
<script type="text/babel">
import ElInputNumber from 'element-ui/packages/input-number';
import SliderButton from './button.vue';
import SliderMarker from './marker';
import Emitter from 'element-ui/src/mixins/emitter';
export default {
name: 'ElSlider',
mixins: [Emitter],
inject: {
elForm: {
default: ''
}
},
props: {
min: {
type: Number,
default: 0
},
max: {
type: Number,
default: 100
},
step: {
type: Number,
default: 1
},
value: {
type: [Number, Array],
default: 0
},
showInput: {
type: Boolean,
default: false
},
showInputControls: {
type: Boolean,
default: true
},
inputSize: {
type: String,
default: 'small'
},
showStops: {
type: Boolean,
default: false
},
showTooltip: {
type: Boolean,
default: true
},
formatTooltip: Function,
disabled: {
type: Boolean,
default: false
},
range: {
type: Boolean,
default: false
},
vertical: {
type: Boolean,
default: false
},
height: {
type: String
},
debounce: {
type: Number,
default: 300
},
label: {
type: String
},
tooltipClass: String,
marks: Object
},
components: {
ElInputNumber,
SliderButton,
SliderMarker
},
data() {
return {
firstValue: null,
secondValue: null,
oldValue: null,
dragging: false,
sliderSize: 1
};
},
watch: {
value(val, oldVal) {
if (this.dragging ||
Array.isArray(val) &&
Array.isArray(oldVal) &&
val.every((item, index) => item === oldVal[index])) {
return;
}
this.setValues();
},
dragging(val) {
if (!val) {
this.setValues();
}
},
firstValue(val) {
if (this.range) {
this.$emit('input', [this.minValue, this.maxValue]);
} else {
this.$emit('input', val);
}
},
secondValue() {
if (this.range) {
this.$emit('input', [this.minValue, this.maxValue]);
}
},
min() {
this.setValues();
},
max() {
this.setValues();
}
},
methods: {
valueChanged() {
if (this.range) {
return ![this.minValue, this.maxValue]
.every((item, index) => item === this.oldValue[index]);
} else {
return this.value !== this.oldValue;
}
},
setValues() {
if (this.min > this.max) {
console.error('[Element Error][Slider]min should not be greater than max.');
return;
}
const val = this.value;
if (this.range && Array.isArray(val)) {
if (val[1] < this.min) {
this.$emit('input', [this.min, this.min]);
} else if (val[0] > this.max) {
this.$emit('input', [this.max, this.max]);
} else if (val[0] < this.min) {
this.$emit('input', [this.min, val[1]]);
} else if (val[1] > this.max) {
this.$emit('input', [val[0], this.max]);
} else {
this.firstValue = val[0];
this.secondValue = val[1];
if (this.valueChanged()) {
this.dispatch('ElFormItem', 'el.form.change', [this.minValue, this.maxValue]);
this.oldValue = val.slice();
}
}
} else if (!this.range && typeof val === 'number' && !isNaN(val)) {
if (val < this.min) {
this.$emit('input', this.min);
} else if (val > this.max) {
this.$emit('input', this.max);
} else {
this.firstValue = val;
if (this.valueChanged()) {
this.dispatch('ElFormItem', 'el.form.change', val);
this.oldValue = val;
}
}
}
},
setPosition(percent) {
const targetValue = this.min + percent * (this.max - this.min) / 100;
if (!this.range) {
this.$refs.button1.setPosition(percent);
return;
}
let button;
if (Math.abs(this.minValue - targetValue) < Math.abs(this.maxValue - targetValue)) {
button = this.firstValue < this.secondValue ? 'button1' : 'button2';
} else {
button = this.firstValue > this.secondValue ? 'button1' : 'button2';
}
this.$refs[button].setPosition(percent);
},
onSliderClick(event) {
if (this.sliderDisabled || this.dragging) return;
this.resetSize();
if (this.vertical) {
const sliderOffsetBottom = this.$refs.slider.getBoundingClientRect().bottom;
this.setPosition((sliderOffsetBottom - event.clientY) / this.sliderSize * 100);
} else {
const sliderOffsetLeft = this.$refs.slider.getBoundingClientRect().left;
this.setPosition((event.clientX - sliderOffsetLeft) / this.sliderSize * 100);
}
this.emitChange();
},
resetSize() {
if (this.$refs.slider) {
this.sliderSize = this.$refs.slider[`client${ this.vertical ? 'Height' : 'Width' }`];
}
},
emitChange() {
this.$nextTick(() => {
this.$emit('change', this.range ? [this.minValue, this.maxValue] : this.value);
});
},
getStopStyle(position) {
return this.vertical ? { 'bottom': position + '%' } : { 'left': position + '%' };
}
},
computed: {
stops() {
if (!this.showStops || this.min > this.max) return [];
if (this.step === 0) {
process.env.NODE_ENV !== 'production' &&
console.warn('[Element Warn][Slider]step should not be 0.');
return [];
}
const stopCount = (this.max - this.min) / this.step;
const stepWidth = 100 * this.step / (this.max - this.min);
const result = [];
for (let i = 1; i < stopCount; i++) {
result.push(i * stepWidth);
}
if (this.range) {
return result.filter(step => {
return step < 100 * (this.minValue - this.min) / (this.max - this.min) ||
step > 100 * (this.maxValue - this.min) / (this.max - this.min);
});
} else {
return result.filter(step => step > 100 * (this.firstValue - this.min) / (this.max - this.min));
}
},
markList() {
if (!this.marks) {
return [];
}
const marksKeys = Object.keys(this.marks);
return marksKeys.map(parseFloat)
.sort((a, b) => a - b)
.filter(point => point <= this.max && point >= this.min)
.map(point => ({
point,
position: (point - this.min) * 100 / (this.max - this.min),
mark: this.marks[point]
}));
},
minValue() {
return Math.min(this.firstValue, this.secondValue);
},
maxValue() {
return Math.max(this.firstValue, this.secondValue);
},
barSize() {
return this.range
? `${ 100 * (this.maxValue - this.minValue) / (this.max - this.min) }%`
: `${ 100 * (this.firstValue - this.min) / (this.max - this.min) }%`;
},
barStart() {
return this.range
? `${ 100 * (this.minValue - this.min) / (this.max - this.min) }%`
: '0%';
},
precision() {
let precisions = [this.min, this.max, this.step].map(item => {
let decimal = ('' + item).split('.')[1];
return decimal ? decimal.length : 0;
});
return Math.max.apply(null, precisions);
},
runwayStyle() {
return this.vertical ? { height: this.height } : {};
},
barStyle() {
return this.vertical
? {
height: this.barSize,
bottom: this.barStart
} : {
width: this.barSize,
left: this.barStart
};
},
sliderDisabled() {
return this.disabled || (this.elForm || {}).disabled;
}
},
mounted() {
let valuetext;
if (this.range) {
if (Array.isArray(this.value)) {
this.firstValue = Math.max(this.min, this.value[0]);
this.secondValue = Math.min(this.max, this.value[1]);
} else {
this.firstValue = this.min;
this.secondValue = this.max;
}
this.oldValue = [this.firstValue, this.secondValue];
valuetext = `${this.firstValue}-${this.secondValue}`;
} else {
if (typeof this.value !== 'number' || isNaN(this.value)) {
this.firstValue = this.min;
} else {
this.firstValue = Math.min(this.max, Math.max(this.min, this.value));
}
this.oldValue = this.firstValue;
valuetext = this.firstValue;
}
this.$el.setAttribute('aria-valuetext', valuetext);
// label screen reader
this.$el.setAttribute('aria-label', this.label ? this.label : `slider between ${this.min} and ${this.max}`);
this.resetSize();
window.addEventListener('resize', this.resetSize);
},
beforeDestroy() {
window.removeEventListener('resize', this.resetSize);
}
};
</script>
自定义封装
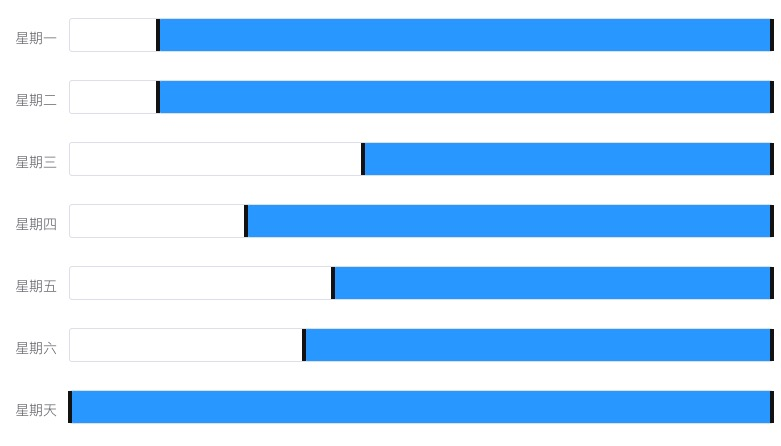
[需求分析] 由于项目中用到的是日期模板的显示,因而主体拖拽事件等可直接使用el-slider,样式方面可以自定义设置;其次就是日期的显示,el-slider的字段设置为数字,因而需要将日期等进行转换,此时用到moment.js这个库,方便统一处理
[目录分析] 主目录下还是vue的组件,将日期类的方法抽离到了utils下的format.js中
[解决方案] 主要还是template、script、style三部分
template代码:
<template>
<div class="range-container">
<el-slider
v-model="s"
:format-tooltip="formatTooltip"
:max="24"
:step='1'
range
@change='handleChange'
>
</el-slider>
</div>
</template>
script代码:
<script>
// 工具函数
import { formatHoursMinutes } from '@/utils/format';
export default {
data() {
return {
s: [this.start,this.isFullDay(this.end)],
formatHoursMinutes: formatHoursMinutes
}
},
props: {
start: {
default: 0,
type: Number
},
end: {
default: 24,
type: Number
},
week: {
default: 1,
type: String
}
},
methods: {
isFullDay(val) {
if(val === 0) {
return 24
} else {
return Number(val)
}
},
formatTooltip(val) {
// 进行格式转换
let n = parseInt(val / 1);
return this.formatHoursMinutes(n)
},
handleChange(val) {
const [start,end] = val;
const week = this.week;
this.$emit('changeTemplate',{start,end,week})
}
}
}
</script>
style代码:
<style lang='scss'>
@import '@/styles/element-variables.scss';
.range-container {
.el-slider {
.el-slider__runway {
height: 32px;
margin-top: 0;
margin-bottom: 0 !important;
background-color: #FFFFFF;
border: 1px solid #DCDFE6;
.el-slider__bar {
height: 32px;
}
.el-slider__button-wrapper {
top: 0;
height: 32px;
.el-slider__button {
width: 4px;
height: 32px;
border-radius: 0;
background: #121212;
border: none;
}
}
.el-slider__stop {
width: 1px;
height: 31px;
border-radius: 0;
background-color: #DCDFE6;
}
.el-slider__marks-text {
color: #717171;
margin-top: 0;
transform: translateX(-115%);
}
}
}
}
</style>
工具函数:
import moment from 'moment';
// 修改星期对应数值
export function formatWeek(val) {
switch (val) {
case '1':
return '星期一'
break;
case '2':
return '星期二'
break;
case '3':
return '星期三'
break;
case '4':
return '星期四'
break;
case '5':
return '星期五'
break;
case '6':
return '星期六'
break;
case '7':
return '星期天'
break;
default:
break;
}
};
// 修改数值对应小时格式
export function formatHours(val) {
return moment(val,'H').hours();
};
// 修改数值对应小时分钟格式
export function formatHoursMinutes(val) {
return moment().hour(val).minute(0).second(0).format("HH:mm");
};
// 修改数值对应小时分钟格式
export function formatHoursMinutesSeconds(val) {
return moment().hour(val).minute(0).second(0).format("HH:mm:ss");
};
封装range组件常见方法
封装类range组件主要就是三块: 1、展示:主要就是进度条、滑块、显示,需要考虑行为层的接入及展示; 2、行为:主要就是mouse、touch、drag行为的封装,一般来说会封成一个函数库,不管是原生封装、jQuery封装还是直接引用别人封装好的库,最后暴露出去都需要考虑展示层接入的范围、类型等; 3、扩展:封装的组件提供一个很好的扩展性有利于引入及修改
总结
在项目中常常需要根据需要进行组件的二次封装,在封装过程中,对组件库的理解以及对场景的扩展把握可以更好的避免组件的更改等其他因素的产生,提高功效,同时借鉴其他思路也是很好的方法,对于有志于从0封装自己一套组件库的同学,还需要掌握库的打包、环境配置、扩展以及社区的考虑,可以参考这篇文章从0到1教你搭建前端团队的组件系统(高级进阶必备),对于只是业务的二次封装,基于对源码的分析也能很好的提升自身的封装能力。